JQuery Handbook
jQuery is a JavaScript Library.
jQuery greatly simplifies JavaScript programming.
jQuery Examples
jQuery Selector
jQuery Introduction
What is jQuery?
jQuery is a lightweight, "write less, do more", JavaScript library.
The purpose of jQuery is to make it much easier to use JavaScript on your website.
jQuery takes a lot of common tasks that require many lines of JavaScript code to accomplish, and wraps them into methods that you can call with a single line of code.
jQuery also simplifies a lot of the complicated things from JavaScript, like AJAX calls and DOM manipulation.
The jQuery library contains the following features:
- HTML/DOM manipulation
- CSS manipulation
- HTML event methods
- Effects and animations
- AJAX
- Utilities
Tip: In addition, jQuery has plugins for almost any task out there.
Adding jQuery to Your Web Pages
There are several ways to start using jQuery on your web site. You can:
- Download the jQuery library from jQuery.com
- Include jQuery from a CDN, like Google
Downloading jQuery
There are two versions of jQuery available for downloading:
- Production version - this is for your live website because it has been minified and compressed
- Development version - this is for testing and development (uncompressed and readable code)
Both versions can be downloaded from jQuery.com.
The jQuery library is a single JavaScript file, and you reference it with the HTML <script>
tag (notice that the <script>
tag should be inside the <head>
section):
<head>
<script src="jquery-3.7.1.min.js"></script>
</head>
Tip: Place the downloaded file in the same directory as the pages where you wish to use it.
jQuery CDN
If you don't want to download and host jQuery yourself, you can include it from a CDN (Content Delivery Network).
Google is an example of someone who host jQuery:
Google CDN:
<head>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.7.1/jquery.min.js"></script>
</head>
Functions In a Separate File
If your website contains a lot of pages, and you want your jQuery functions to be easy to maintain, you can put your jQuery functions in a separate .js file.
When we demonstrate jQuery in this tutorial, the functions are added directly into the <head>
section. However, sometimes it is preferable to place them in a separate file, like this (use the src attribute to refer to the .js file):
<head>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.7.1/jquery.min.js"></script>
<script src="my_jquery_functions.js"></script>
</head>
jQuery Syntax
With jQuery you select (query) HTML elements and perform "actions" on them.
jQuery Syntax
The jQuery syntax is tailor-made for selecting HTML elements and performing some action on the element(s).
Basic syntax is: $(selector).action()
- A $ sign to define/access jQuery
- A (selector) to "query (or find)" HTML elements
- A jQuery action() to be performed on the element(s)
Examples:
$(this).hide()
- hides the current element.
$("p").hide()
- hides all <p>
elements.
$(".test").hide()
- hides all elements with class="test".
$("#test").hide()
- hides the element with id="test".
The Document Ready Event
You might have noticed that all jQuery methods in our examples, are inside a document ready event:
$(document).ready(function(){
_// jQuery methods go here..._
});
This is to prevent any jQuery code from running before the document is finished loading (is ready).
It is good practice to wait for the document to be fully loaded and ready before working with it. This also allows you to have your JavaScript code before the body of your document, in the head section.
Here are some examples of actions that can fail if methods are run before the document is fully loaded:
- Trying to hide an element that is not created yet
- Trying to get the size of an image that is not loaded yet
Tip: The jQuery team has also created an even shorter method for the document ready event:
$(function(){
_// jQuery methods go here..._
});
Use the syntax you prefer. We think that the document ready event is easier to understand when reading the code.
jQuery Selectors
jQuery selectors allow you to select and manipulate HTML element(s).
jQuery selectors are used to "find" (or select) HTML elements based on their name
, id
, classes
, types
, attributes
, values
of attributes and much more. It's based on the existing CSS Selectors, and in addition, it has some own custom selectors.
All selectors in jQuery start with the dollar sign and parentheses: $()
.
The element
Selector
The jQuery element selector selects elements based on the element name.
You can select all <p>
elements on a page like this:
$("p")
Example
$(document).ready(function(){
$("button").click(function(){
$("p").hide();
});
});
The #id
Selector
The jQuery #id
selector uses the id attribute of an HTML tag to find the specific element.
An id
should be unique within a page, so you should use the #id
selector when you want to find a single, unique element.
To find an element with a specific id
, write a hash character, followed by the id of the HTML element:
$("#test")
Example
When a user clicks on a button, the element with id="test" will be hidden:
$(document).ready(function(){
$("button").click(function(){
$("#test").hide();
});
});
The .class
Selector
The jQuery .class
selector finds elements with a specific class.
To find elements with a specific class, write a period character, followed by the name of the class:
$(".test")
Example
When a user clicks on a button, the elements with class="test" will be hidden:
$(document).ready(function(){
$("button").click(function(){
$(".test").hide();
});
});
More Examples of jQuery Selectors
Use our jQuery Selector Tester to demonstrate the different selectors.
jQuery Selectors References
Selector | Example | Selects |
---|---|---|
* | $("*") | All elements |
#id | $("#lastname") | The element with id="lastname" |
.class | $(".intro") | All elements with class="intro" |
.class,.class | $(".intro,.demo") | All elements with the class "intro" or "demo" |
element | $("p") | All <p> elements |
el1,el2,el3 | $("h1,div,p") | All <h1> , <div> and <p> elements |
:first | $("p:first") | The first <p> element |
:last | $("p:last") | The last <p> element |
:even | $("tr:even") | All even <tr> elements |
:odd | $("tr:odd") | All odd <tr> elements |
:first-child | $("p:first-child") | All <p> elements that are the first child of their parent |
:first-of-type | $("p:first-of-type") | All <p> elements that are the first <p> element of their parent |
:last-child | $("p:last-child") | All <p> elements that are the last child of their parent |
:last-of-type | $("p:last-of-type") | All <p> elements that are the last <p> element of their parent |
:nth-child(n) | $("p:nth-child(2)") | All <p> elements that are the 2nd child of their parent |
:nth-last-child(n) | $("p:nth-last-child(2)") | All <p> elements that are the 2nd child of their parent, counting from the last child |
:nth-of-type(n) | $("p:nth-of-type(2)") | All <p> elements that are the 2nd <p> element of their parent |
:nth-last-of-type(n) | $("p:nth-last-of-type(2)") | All <p> elements that are the 2nd <p> element of their parent, counting from the last child |
:only-child | $("p:only-child") | All <p> elements that are the only child of their parent |
:only-of-type | $("p:only-of-type") | All <p> elements that are the only child, of its type, of their parent |
parent > child | $("div > p") | All <p> elements that are a direct child of a <div> element |
parent descendant | $("div p") | All <p> elements that are descendants of a <div> element |
element + next | $("div + p") | The <p> element that are next to each <div> elements |
element ~ siblings | $("div ~ p") | All <p> elements that appear after the <div> element |
:eq(index) | $("ul li:eq(3)") | The fourth element in a list (index starts at 0) |
:gt(no) | $("ul li:gt(3)") | List elements with an index greater than 3 |
:lt(no) | $("ul li:lt(3)") | List elements with an index less than 3 |
:not(selector) | $("input:not(:empty)") | All input elements that are not empty |
:header | $(":header") | All header elements <h1> , <h2> ... |
:animated | $(":animated") | All animated elements |
:focus | $(":focus") | The element that currently has focus |
:contains(text) | $(":contains('Hello')") | All elements which contains the text "Hello" |
:has(selector) | $("div:has(p)") | All <div> elements that have a <p> element |
:empty | $(":empty") | All elements that are empty |
:parent | $(":parent") | All elements that are a parent of another element |
:hidden | $("p:hidden") | All hidden <p> elements |
:visible | $("table:visible") | All visible tables |
:root | $(":root") | The document's root element |
:lang(language) | $("p:lang(de)") | All <p> elements with a lang attribute value starting with "de" |
[attribute] | $("[href]") | All elements with a href attribute |
[attribute=value] | $("[href='default.htm']") | All elements with a href attribute value equal to "default.htm" |
[attribute!=value] | $("[href!='default.htm']") | All elements with a href attribute value not equal to "default.htm" |
[attribute$=value] | $("[href$='.jpg']") | All elements with a href attribute value ending with ".jpg" |
[attribute|=value] | $("[title|='Tomorrow']") | All elements with a title attribute value equal to 'Tomorrow', or starting with 'Tomorrow' followed by a hyphen |
[attribute^=value] | $("[title^='Tom']") | All elements with a title attribute value starting with "Tom" |
[attribute~=value] | $("[title~='hello']") | All elements with a title attribute value containing the specific word "hello" |
[attribute*=value] | $("[title*='hello']") | All elements with a title attribute value containing the word "hello" |
:input | $(":input") | All input elements |
:text | $(":text") | All input elements with type="text" |
:password | $(":password") | All input elements with type="password" |
:radio | $(":radio") | All input elements with type="radio" |
:checkbox | $(":checkbox") | All input elements with type="checkbox" |
:submit | $(":submit") | All input elements with type="submit" |
:reset | $(":reset") | All input elements with type="reset" |
:button | $(":button") | All input elements with type="button" |
:image | $(":image") | All input elements with type="image" |
:file | $(":file") | All input elements with type="file" |
:enabled | $(":enabled") | All enabled input elements |
:disabled | $(":disabled") | All disabled input elements |
:selected | $(":selected") | All selected input elements |
:checked | $(":checked") | All checked input elements |
jQuery Event Methods
What are Events?
All the different visitors' actions that a web page can respond to are called events.
An event represents the precise moment when something happens.
Examples:
- moving a mouse over an element
- selecting a radio button
- clicking on an element
The term "fires/fired" is often used with events. Example: "The keypress event is fired, the moment you press a key".
Here are some common DOM events:
Mouse Events | Keyboard Events | Form Events | Document/Window Events |
---|---|---|---|
click | keypress | submit | load |
dblclick | keydown | change | resize |
mouseenter | keyup | focus | scroll |
mouseleave | blur | unload |
jQuery Syntax For Event Methods
In jQuery, most DOM events have an equivalent jQuery method.
To assign a click event to all paragraphs on a page, you can do this:
$("p").click();
The next step is to define what should happen when the event fires. You must pass a function to the event:
$("p").click(function(){
// action goes here!!
});
Commonly Used jQuery Event Methods
$(document).ready()
The $(document).ready()
method allows us to execute a function when the document is fully loaded. This event is already explained in the jQuery Syntax chapter.
click()
The click()
method attaches an event handler function to an HTML element.
The function is executed when the user clicks on the HTML element.
The following example says: When a click event fires on a <p>
element; hide the current <p>
element:
$("p").click(function(){
$(this).hide();
});
dblclick()
The dblclick()
method attaches an event handler function to an HTML element.
The function is executed when the user double-clicks on the HTML element:
$("p").dblclick(function(){
$(this).hide();
});
mouseenter()
The mouseenter()
method attaches an event handler function to an HTML element.
The function is executed when the mouse pointer enters the HTML element:
$("#p1").mouseenter(function(){
alert("You entered p1!");
});
mouseleave()
The mouseleave()
method attaches an event handler function to an HTML element.
The function is executed when the mouse pointer leaves the HTML element:
$("#p1").mouseleave(function(){
alert("Bye! You now leave p1!");
});
mousedown()
The mousedown()
method attaches an event handler function to an HTML element.
The function is executed, when the left, middle or right mouse button is pressed down, while the mouse is over the HTML element:
$("#p1").mousedown(function(){
alert("Mouse down over p1!");
});
mouseup()
The mouseup()
method attaches an event handler function to an HTML element.
The function is executed, when the left, middle or right mouse button is released, while the mouse is over the HTML element:
$("#p1").mouseup(function(){
alert("Mouse up over p1!");
});
hover()
The hover()
method takes two functions and is a combination of the mouseenter()
and mouseleave()
methods.
The first function is executed when the mouse enters the HTML element, and the second function is executed when the mouse leaves the HTML element:
$("#p1").hover(function(){
alert("You entered p1!");
},
function(){
alert("Bye! You now leave p1!");
});
focus()
The focus()
method attaches an event handler function to an HTML form field.
The function is executed when the form field gets focus:
$("input").focus(function(){
$(this).css("background-color", "#cccccc");
});
blur()
The blur()
method attaches an event handler function to an HTML form field.
The function is executed when the form field loses focus:
$("input").blur(function(){
$(this).css("background-color", "#ffffff");
});
The on() Method
The on()
method attaches one or more event handlers for the selected elements.
Attach a click event to a <p>
element:
Example
$("p").on("click", function(){
$(this).hide();
});
Attach multiple event handlers to a <p>
element:
Example
$("p").on({
mouseenter: function(){
$(this).css("background-color", "lightgray");
},
mouseleave: function(){
$(this).css("background-color", "lightblue");
},
click: function(){
$(this).css("background-color", "yellow");
}
});
jQuery Events References
The following table lists all the jQuery methods used to handle events.
Method / Property | Description |
---|---|
bind() | Deprecated in version 3.0. Use the on() method instead. Attaches event handlers to elements |
blur() | Attaches/Triggers the blur event |
change() | Attaches/Triggers the change event |
click() | Attaches/Triggers the click event |
dblclick() | Attaches/Triggers the double click event |
delegate() | Deprecated in version 3.0. Use the on() method instead. Attaches a handler to current, or future, specified child elements of the matching elements |
die() | Removed in version 1.9. Removes all event handlers added with the live() method |
error() | Removed in version 3.0. Attaches/Triggers the error event |
event.currentTarget | The current DOM element within the event bubbling phase |
event.data | Contains the optional data passed to an event method when the current executing handler is bound |
event.delegateTarget | Returns the element where the currently-called jQuery event handler was attached |
event.isDefaultPrevented() | Returns whether event.preventDefault() was called for the event object |
event.isImmediatePropagationStopped() | Returns whether event.stopImmediatePropagation() was called for the event object |
event.isPropagationStopped() | Returns whether event.stopPropagation() was called for the event object |
event.namespace | Returns the namespace specified when the event was triggered |
event.pageX | Returns the mouse position relative to the left edge of the document |
event.pageY | Returns the mouse position relative to the top edge of the document |
event.preventDefault() | Prevents the default action of the event |
event.relatedTarget | Returns which element being entered or exited on mouse movement |
event.result | Contains the last/previous value returned by an event handler triggered by the specified event |
event.stopImmediatePropagation() | Prevents other event handlers from being called |
event.stopPropagation() | Prevents the event from bubbling up the DOM tree, preventing any parent handlers from being notified of the event |
event.target | Returns which DOM element triggered the event |
event.timeStamp | Returns the number of milliseconds since January 1, 1970, when the event is triggered |
event.type | Returns which event type was triggered |
event.which | Returns which keyboard key or mouse button was pressed for the event |
focus() | Attaches/Triggers the focus event |
focusin() | Attaches an event handler to the focusin event |
focusout() | Attaches an event handler to the focusout event |
hover() | Attaches two event handlers to the hover event |
keydown() | Attaches/Triggers the keydown event |
keypress() | Attaches/Triggers the keypress event |
keyup() | Attaches/Triggers the keyup event |
live() | Removed in version 1.9. Adds one or more event handlers to current, or future, selected elements |
load() | Removed in version 3.0. Attaches an event handler to the load event |
mousedown() | Attaches/Triggers the mousedown event |
mouseenter() | Attaches/Triggers the mouseenter event |
mouseleave() | Attaches/Triggers the mouseleave event |
mousemove() | Attaches/Triggers the mousemove event |
mouseout() | Attaches/Triggers the mouseout event |
mouseover() | Attaches/Triggers the mouseover event |
mouseup() | Attaches/Triggers the mouseup event |
off() | Removes event handlers attached with the on() method |
on() | Attaches event handlers to elements |
one() | Adds one or more event handlers to selected elements. This handler can only be triggered once per element |
$.proxy() | Takes an existing function and returns a new one with a particular context |
ready() | Specifies a function to execute when the DOM is fully loaded |
resize() | Attaches/Triggers the resize event |
scroll() | Attaches/Triggers the scroll event |
select() | Attaches/Triggers the select event |
submit() | Attaches/Triggers the submit event |
toggle() | Removed in version 1.9. Attaches two or more functions to toggle between for the click event |
trigger() | Triggers all events bound to the selected elements |
triggerHandler() | Triggers all functions bound to a specified event for the selected elements |
unbind() | Deprecated in version 3.0. Use the off() method instead. Removes an added event handler from selected elements |
undelegate() | Deprecated in version 3.0. Use the off() method instead. Removes an event handler to selected elements, now or in the future |
unload() | Removed in version 3.0. Use the on() or trigger() method instead. Attaches an event handler to the unload event |
# jQuery Effects
jQuery Fading Methods
With jQuery you can fade an element in and out of visibility.
jQuery has the following fade methods:
fadeIn()
fadeOut()
fadeToggle()
fadeTo()
jQuery Sliding Methods
With jQuery you can create a sliding effect on elements.
jQuery has the following slide methods:
slideDown()
slideUp()
slideToggle()
jQuery Animations - The animate() Method
The jQuery animate()
method is used to create custom animations.
jQuery stop() Method
The jQuery stop()
method is used to stop an animation or effect before it is finished.
jQuery Callback Functions
JavaScript statements are executed line by line. However, with effects, the next line of code can be run even though the effect is not finished. This can create errors.
To prevent this, you can create a callback function.
A callback function is executed after the current effect is finished.
Typical syntax: $(_selector_).hide(_speed,callback_);
Examples
The example below has a callback parameter that is a function that will be executed after the hide effect is completed:
Example with Callback
$("button").click(function(){
$("p").hide("slow", function(){
alert("The paragraph is now hidden");
});
});
The example below has no callback parameter, and the alert box will be displayed before the hide effect is completed:
Example without Callback
$("button").click(function(){
$("p").hide(1000);
alert("The paragraph is now hidden");
});
jQuery - Chaining
With jQuery, you can chain together actions/methods.
Chaining allows us to run multiple jQuery methods (on the same element) within a single statement.
jQuery Method Chaining
Until now we have been writing jQuery statements one at a time (one after the other).
However, there is a technique called chaining, that allows us to run multiple jQuery commands, one after the other, on the same element(s).
Tip: This way, browsers do not have to find the same element(s) more than once.
To chain an action, you simply append the action to the previous action.
The following example chains together the css()
, slideUp()
, and slideDown()
methods. The "p1" element first changes to red, then it slides up, and then it slides down:
Example
$("#p1").css("color", "red").slideUp(2000).slideDown(2000);
We could also have added more method calls if needed.
Tip: When chaining, the line of code could become quite long. However, jQuery is not very strict on the syntax; you can format it like you want, including line breaks and indentations.
This also works just fine:
Example
$("#p1").css("color", "red")
.slideUp(2000)
.slideDown(2000);
jQuery throws away extra whitespace and executes the lines above as one long line of code.
jQuery HTML
jQuery DOM Manipulation
DOM = Document Object Model
Get/Set Content
Three simple, but useful, jQuery methods for DOM manipulation are:
text()
- Sets or returns the text content of selected elementshtml()
- Sets or returns the content of selected elements (including HTML markup)val()
- Sets or returns the value of form fields
Add New HTML Content
We will look at four jQuery methods that are used to add new content:
append()
- Inserts content at the end of the selected elementsprepend()
- Inserts content at the beginning of the selected elementsafter()
- Inserts content after the selected elementsbefore()
- Inserts content before the selected elements
Remove Elements/Content
To remove elements and content, there are mainly two jQuery methods:
remove()
- Removes the selected element (and its child elements)empty()
- Removes the child elements from the selected element
jQuery Manipulating CSS
jQuery has several methods for CSS manipulation. We will look at the following methods:
addClass()
- Adds one or more classes to the selected elementsremoveClass()
- Removes one or more classes from the selected elementstoggleClass()
- Toggles between adding/removing classes from the selected elementscss()
- Sets or returns the style attribute
jQuery css() Method
Return a CSS Property
css("_propertyname_");
$("p").css("background-color");
Set a CSS Property
css("_propertyname_","_value_");
$("p").css("background-color", "yellow");
Set Multiple CSS Properties
css({"_propertyname_":"_value_","_propertyname_":"_value_",...});
$("p").css({"background-color": "yellow", "font-size": "200%"});
jQuery HTML / CSS Reference
The following table lists all the methods used to manipulate the HTML and CSS.
The methods below work for both HTML and XML documents. Exception: the html() method.
Method | Description |
---|---|
addClass() | Adds one or more class names to selected elements |
after() | Inserts content after selected elements |
append() | Inserts content at the end of selected elements |
appendTo() | Inserts HTML elements at the end of selected elements |
attr() | Sets or returns attributes/values of selected elements |
before() | Inserts content before selected elements |
clone() | Makes a copy of selected elements |
css() | Sets or returns one or more style properties for selected elements |
detach() | Removes selected elements (keeps data and events) |
empty() | Removes all child nodes and content from selected elements |
hasClass() | Checks if any of the selected elements have a specified class name |
height() | Sets or returns the height of selected elements |
html() | Sets or returns the content of selected elements |
innerHeight() | Returns the height of an element (includes padding, but not border) |
innerWidth() | Returns the width of an element (includes padding, but not border) |
insertAfter() | Inserts HTML elements after selected elements |
insertBefore() | Inserts HTML elements before selected elements |
offset() | Sets or returns the offset coordinates for selected elements (relative to the document) |
offsetParent() | Returns the first positioned parent element |
outerHeight() | Returns the height of an element (includes padding and border) |
outerWidth() | Returns the width of an element (includes padding and border) |
position() | Returns the position (relative to the parent element) of an element |
prepend() | Inserts content at the beginning of selected elements |
prependTo() | Inserts HTML elements at the beginning of selected elements |
prop() | Sets or returns properties/values of selected elements |
remove() | Removes the selected elements (including data and events) |
removeAttr() | Removes one or more attributes from selected elements |
removeClass() | Removes one or more classes from selected elements |
removeProp() | Removes a property set by the prop() method |
replaceAll() | Replaces selected elements with new HTML elements |
replaceWith() | Replaces selected elements with new content |
scrollLeft() | Sets or returns the horizontal scrollbar position of selected elements |
scrollTop() | Sets or returns the vertical scrollbar position of selected elements |
text() | Sets or returns the text content of selected elements |
toggleClass() | Toggles between adding/removing one or more classes from selected elements |
unwrap() | Removes the parent element of the selected elements |
val() | Sets or returns the value attribute of the selected elements (for form elements) |
width() | Sets or returns the width of selected elements |
wrap() | Wraps HTML element(s) around each selected element |
wrapAll() | Wraps HTML element(s) around all selected elements |
wrapInner() | Wraps HTML element(s) around the content of each selected element |
jQuery Dimension Methods
jQuery has several important methods for working with dimensions:
width()
height()
innerWidth()
innerHeight()
outerWidth()
outerHeight()
jQuery Dimensions
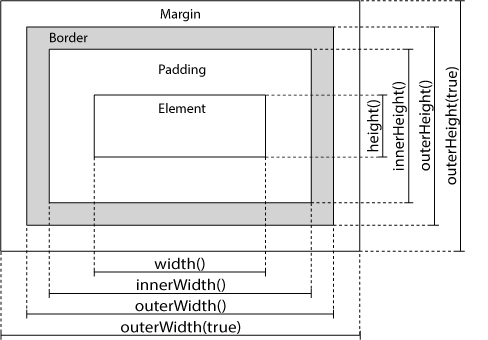
Example
The following example sets the width and height of a specified <div>
element:
$("button").click(function(){
$("#div1").width(500).height(500);
});
jQuery Traversing
What is Traversing?
jQuery traversing, which means "move through", are used to "find" (or select) HTML elements based on their relation to other elements. Start with one selection and move through that selection until you reach the elements you desire.
The image below illustrates an HTML page as a tree (DOM tree). With jQuery traversing, you can easily move up (ancestors), down (descendants) and sideways (siblings) in the tree, starting from the selected (current) element. This movement is called traversing - or moving through - the DOM tree.
Illustration explained:
- The
<div>
element is the parent of<ul>
, and an ancestor of everything inside of it - The
<ul>
element is the parent of both<li>
elements, and a child of<div>
- The left
<li>
element is the parent of<span>
, child of<ul>
and a descendant of<div>
- The
<span>
element is a child of the left<li>
and a descendant of<ul>
and<div>
- The two
<li>
elements are siblings (they share the same parent) - The right
<li>
element is the parent of<b>
, child of<ul>
and a descendant of<div>
- The
<b>
element is a child of the right<li>
and a descendant of<ul>
and<div>
Note:
- An ancestor is a parent, grandparent, great-grandparent, and so on.
- A descendant is a child, grandchild, great-grandchild, and so on.
- Siblings share the same parent.
jQuery Traversing - Ancestors
With jQuery you can traverse up the DOM tree to find ancestors of an element.
An ancestor is a parent, grandparent, great-grandparent, and so on.
Traversing Up the DOM Tree
Three useful jQuery methods for traversing up the DOM tree are:
parent() | $("span").parent() |
---|---|
parents() | $("span").parents() |
parentsUntil() | $("span").parentsUntil("div") |
jQuery Traversing - Descendants
With jQuery you can traverse down the DOM tree to find descendants of an element.
A descendant is a child, grandchild, great-grandchild, and so on.
Traversing Down the DOM Tree
children() | $("div").children() |
---|---|
find() | $("div").find("span") |
jQuery Traversing - Siblings
With jQuery you can traverse sideways in the DOM tree to find siblings of an element.
Siblings share the same parent.
Traversing Sideways in The DOM Tree
siblings()
next()
nextAll()
nextUntil()
prev()
prevAll()
prevUntil()
jQuery Traversing - Filtering
The first(), last(), eq(), filter() and not() Methods
The most basic filtering methods are first()
, last()
and eq()
, which allow you to select a specific element based on its position in a group of elements.
Other filtering methods, like filter()
and not()
allow you to select elements that match, or do not match, a certain criteria.
jQuery Traversing Reference
Method | Description |
---|---|
add() | Adds elements to the set of matched elements |
addBack() | Adds the previous set of elements to the current set |
andSelf() | Deprecated in version 1.8. An alias for addBack() |
children() | Returns all direct children of the selected element |
closest() | Returns the first ancestor of the selected element |
contents() | Returns all direct children of the selected element (including text and comment nodes) |
each() | Executes a function for each matched element |
end() | Ends the most recent filtering operation in the current chain, and return the set of matched elements to its previous state |
eq() | Returns an element with a specific index number of the selected elements |
filter() | Reduce the set of matched elements to those that match the selector or pass the function's test |
find() | Returns descendant elements of the selected element |
first() | Returns the first element of the selected elements |
has() | Returns all elements that have one or more elements inside of them |
is() | Checks the set of matched elements against a selector/element/jQuery object, and return true if at least one of these elements matches the given arguments |
last() | Returns the last element of the selected elements |
map() | Passes each element in the matched set through a function, producing a new jQuery object containing the return values |
next() | Returns the next sibling element of the selected element |
nextAll() | Returns all next sibling elements of the selected element |
nextUntil() | Returns all next sibling elements between two given arguments |
not() | Returns elements that do not match a certain criteria |
offsetParent() | Returns the first positioned parent element |
parent() | Returns the direct parent element of the selected element |
parents() | Returns all ancestor elements of the selected element |
parentsUntil() | Returns all ancestor elements between two given arguments |
prev() | Returns the previous sibling element of the selected element |
prevAll() | Returns all previous sibling elements of the selected element |
prevUntil() | Returns all previous sibling elements between two given arguments |
siblings() | Returns all sibling elements of the selected element |
slice() | Reduces the set of matched elements to a subset specified by a range of indices |
jQuery - AJAX Introduction
AJAX is the art of exchanging data with a server, and updating parts of a web page - without reloading the whole page.
What is AJAX?
AJAX = Asynchronous JavaScript and XML.
In short; AJAX is about loading data in the background and display it on the webpage, without reloading the whole page.
What About jQuery and AJAX?
jQuery provides several methods for AJAX functionality.
With the jQuery AJAX methods, you can request text, HTML, XML, or JSON from a remote server using both HTTP Get and HTTP Post - And you can load the external data directly into the selected HTML elements of your web page!
jQuery - AJAX load() Method
jQuery load() Method
The jQuery load()
method is a simple, but powerful AJAX method.
The load()
method loads data from a server and puts the returned data into the selected element.
Syntax:
$(_selector_).load(_URL,data,callback_);
The required URL parameter specifies the URL you wish to load.
The optional data parameter specifies a set of querystring key/value pairs to send along with the request.
The optional callback parameter is the name of a function to be executed after the load()
method is completed.
Here is the content of our example file: "demo_test.txt":
<h2>jQuery and AJAX is FUN!!!</h2>
<p id="p1">This is some text in a paragraph.</p>
The following example loads the content of the file "demo_test.txt" into a specific <div>
element:
Example
$("#div1").load("demo_test.txt");
It is also possible to add a jQuery selector to the URL parameter.
The following example loads the content of the element with id="p1", inside the file "demo_test.txt", into a specific <div>
element:
Example
$("#div1").load("demo_test.txt #p1");
The optional callback parameter specifies a callback function to run when the load()
method is completed. The callback function can have different parameters:
responseTxt
- contains the resulting content if the call succeedsstatusTxt
- contains the status of the callxhr
- contains the XMLHttpRequest object
The following example displays an alert box after the load() method completes. If the load()
method has succeeded, it displays "External content loaded successfully!", and if it fails it displays an error message:
Example
$("button").click(function(){
$("#div1").load("demo_test.txt", function(responseTxt, statusTxt, xhr){
if(statusTxt == "success")
alert("External content loaded successfully!");
if(statusTxt == "error")
alert("Error: " + xhr.status + ": " + xhr.statusText);
});
});
jQuery - AJAX get() and post() Methods
The jQuery get() and post() methods are used to request data from the server with an HTTP GET or POST request.
HTTP Request: GET vs. POST
Two commonly used methods for a request-response between a client and server are: GET and POST.
- GET - Requests data from a specified resource
- POST - Submits data to be processed to a specified resource
GET is basically used for just getting (retrieving) some data from the server. Note: The GET method may return cached data.
POST can also be used to get some data from the server. However, the POST method NEVER caches data, and is often used to send data along with the request.
To learn more about GET and POST, and the differences between the two methods, please read our HTTP Methods GET vs POST chapter.
jQuery $.get() Method
The $.get()
method requests data from the server with an HTTP GET request.
Syntax:
$.get(_URL,callback_);
The required URL parameter specifies the URL you wish to request.
The optional callback parameter is the name of a function to be executed if the request succeeds.
The following example uses the $.get()
method to retrieve data from a file on the server:
Example
$("button").click(function(){
$.get("demo_test.asp", function(data, status){
alert("Data: " + data + "\nStatus: " + status);
});
});
The first parameter of $.get()
is the URL we wish to request ("demo_test.asp").
The second parameter is a callback function. The first callback parameter holds the content of the page requested, and the second callback parameter holds the status of the request.
Tip: Here is how the ASP file looks like ("demo_test.asp"):
<%
response.write("This is some text from an external ASP file.")
%>
jQuery $.post() Method
The $.post()
method requests data from the server using an HTTP POST request.
Syntax:
$.post(_URL,data,callback_);
The required URL parameter specifies the URL you wish to request.
The optional data parameter specifies some data to send along with the request.
The optional callback parameter is the name of a function to be executed if the request succeeds.
The following example uses the $.post()
method to send some data along with the request:
Example
$("button").click(function(){
$.post("demo_test_post.asp",
{
name: "Donald Duck",
city: "Duckburg"
},
function(data, status){
alert("Data: " + data + "\nStatus: " + status);
});
});
The first parameter of $.post()
is the URL we wish to request ("demo_test_post.asp").
Then we pass in some data to send along with the request (name and city).
The ASP script in "demo_test_post.asp" reads the parameters, processes them, and returns a result.
The third parameter is a callback function. The first callback parameter holds the content of the page requested, and the second callback parameter holds the status of the request.
Tip: Here is how the ASP file looks like ("demo_test_post.asp"):
<%
dim fname,city
fname=Request.Form("name")
city=Request.Form("city")
Response.Write("Dear " & fname & ". ")
Response.Write("Hope you live well in " & city & ".")
%>
jQuery AJAX Reference
Method | Description |
---|---|
$.ajax() | Performs an async AJAX request |
$.ajaxPrefilter() | Handle custom Ajax options or modify existing options before each request is sent and before they are processed by $.ajax() |
$.ajaxSetup() | Sets the default values for future AJAX requests |
$.ajaxTransport() | Creates an object that handles the actual transmission of Ajax data |
$.get() | Loads data from a server using an AJAX HTTP GET request |
$.getJSON() | Loads JSON-encoded data from a server using a HTTP GET request |
$.parseJSON() | Deprecated in version 3.0, use JSON.parse() instead. Takes a well-formed JSON string and returns the resulting JavaScript value |
$.getScript() | Loads (and executes) a JavaScript from a server using an AJAX HTTP GET request |
$.param() | Creates a serialized representation of an array or object (can be used as URL query string for AJAX requests) |
$.post() | Loads data from a server using an AJAX HTTP POST request |
ajaxComplete() | Specifies a function to run when the AJAX request completes |
ajaxError() | Specifies a function to run when the AJAX request completes with an error |
ajaxSend() | Specifies a function to run before the AJAX request is sent |
ajaxStart() | Specifies a function to run when the first AJAX request begins |
ajaxStop() | Specifies a function to run when all AJAX requests have completed |
ajaxSuccess() | Specifies a function to run when an AJAX request completes successfully |
load() | Loads data from a server and puts the returned data into the selected element |
serialize() | Encodes a set of form elements as a string for submission |
serializeArray() | Encodes a set of form elements as an array of names and values |
jQuery - Filters
Use jQuery to filter/search for specific elements.
Filter Tables
Perform a case-insensitive search for items in a table:
<script>
$(document).ready(function(){
$("#myInput").on("keyup", function() {
var value = $(this).val().toLowerCase();
$("#myTable tr").filter(function() {
$(this).toggle($(this).text().toLowerCase().indexOf(value) > -1)
});
});
});
</script>
Filter Lists
Perform a case-insensitive search for items in a list:
Filter Anything
Perform a case-insensitive search for text inside a div element: